Attention: Here be dragons
This is the latest
(unstable) version of this documentation, which may document features
not available in or compatible with released stable versions of Godot.
Checking the stable version of the documentation...
创建 Android 插件¶
前言¶
Android插件是通过挖掘Android平台和生态系统提供的功能来扩展Godot引擎功能的强大工具.
移动游戏货币化就是这样一个例子, 因为它所需要的功能和能力并不属于游戏引擎的核心功能集:
分析
应用内购买
收据验证
安装跟踪
广告
视频广告
交叉推广
游戏中的软硬货币
促销代码
A/B 测试
登录
云保存
排行榜和分数
用户支持和反馈
发布到 Facebook、Twitter 等。
推送通知
Android 插件¶
While introduced in Godot 3.2, the Android plugin system got a significant architecture update starting with Godot 3.2.2.
The new plugin system is backward-incompatible with the previous one and in Godot 4.0, the previous system was fully deprecated and removed.
Since we previously did not version the Android plugin systems, the new one is now labelled v1
and is the starting point for the modern Godot Android ecosystem.
作为前提条件, 请确保你了解如何为Android设置一个 自定义构建环境.
Godot Android 插件的核心是一个 Android 归档库(aar 归档文件),其注意事项如下:
该库必须依赖Godot引擎库(
godot-lib.<version>.<status>.aar
). 每一个Godot版本都有一个稳定的版本, 可以在 Godot 下载页面 .库必须在其清单文件中包含一个专门配置的
<meta-data>
标签.
创建 Android 插件¶
前提条件: Android Studio 强烈建议作为创建Android插件的IDE使用. 下面的说明假设你使用的是Android Studio.
按照 这些说明 为你的插件创建一个Android库模块.
将Godot引擎库作为依赖项添加到插件模块:
Download the Godot engine library (
godot-lib.<version>.<status>.aar
) from the Godot download page (e.g:godot-lib.4.0.stable.aar
).按照 这些说明 来添加Godot引擎库作为你的插件的依赖.
在插件模块的
build.gradle
文件中, 将Godot引擎库的依赖行implementation
改为compileOnly
.
在插件模块中新建一个类,扩展自
org.godotengine.godot.plugin.GodotPlugin
。在运行时,会使用这个类来实例化单例对象,供 Godot 引擎来加载、初始化、运行这个插件。更新插件
AndroidManifest.xml
文件:
打开插件
AndroidManifest.xml
文件.如果
<application></application>
不见了, 请添加该标签.在
<application>
标签中,添加一个<meta-data>
标签设置,如下所示:<meta-data android:name="org.godotengine.plugin.v1.[PluginName]" android:value="[plugin.init.ClassFullName]" />其中
PluginName
是插件的名称,plugin.init.ClassFullName
是插件加载类的全称(包+类名).
为你的插件添加剩余的逻辑, 并运行
gradlew build
命令来生成插件的aar
文件. 构建过程中可能会同时生成debug
和release
的aar
文件. 根据你的需要, 只选择一个版本(通常是release
的版本)提供给你的用户.建议
aar
文件名符合以下模式:[PluginName]*.aar
其中PluginName
是PascalCase中的插件名称(例如:GodotPayment.release.aar
).创建一个Godot Android插件配置文件, 帮助系统检测并加载你的插件:
这配置文件必须是
gdap
(例如:MyPlugin.gdap
).配置文件格式如下:
[config] name="MyPlugin" binary_type="local" binary="MyPlugin.aar" [dependencies] local=["local_dep1.aar", "local_dep2.aar"] remote=["example.plugin.android:remote-dep1:0.0.1", "example.plugin.android:remote-dep2:0.0.1"] custom_maven_repos=["https://repo.mycompany.com/maven2"]
config
部分和字段是必须的, 定义如下:
name:插件的名称。
binary_type:可以是
local
或remote
。类型影响 binary 字段。binary:
如果 binary_type 是
local
,那么这应该是插件aar
文件的文件路径。
文件路径可以是相对的(例如:
MyPlugin.aar
), 在这种情况下, 它是相对于res://android/plugins
目录的.它的文件路径可以是绝对路径:
res://some_path/MyPlugin.aar
.如果 binary_type 是
remote
,那么这应该是一个 remote gradle 二进制 (例如:org.godot.example:my-plugin:0.0.0
)的声明。
dependencies
部分和字段是可选的, 定义如下:
local : 包含插件所依赖的本地
.ar
二进制文件的文件路径列表. 与binary
字段类似(当binary_type
为local
时), 本地二进制文件的文件路径可以是相对的或绝对的.remote:包含该插件的远程 Gradle 依赖二进制的列表。
custom_maven_repos:包含一个 URL 列表,指定该插件依赖所需的自定义 maven 仓库。
加载和使用 Android 插件¶
Move the plugin configuration file (e.g: MyPlugin.gdap
) and, if any, its local binary (e.g: MyPlugin.aar
) and dependencies to the Godot project's res://android/plugins
directory.
Godot编辑器会自动解析 res://android/plugins
目录下的所有 .gdap
文件, 并在 Plugins 部分的Android导出预设窗口中显示检测到的可切换插件列表.
In order to allow GDScript to communicate with your Java Singleton, you must annotate your function with @UsedByGodot
. The name called from GDScript must match the function name exactly. There is no coercing snake_case
to camelCase
.
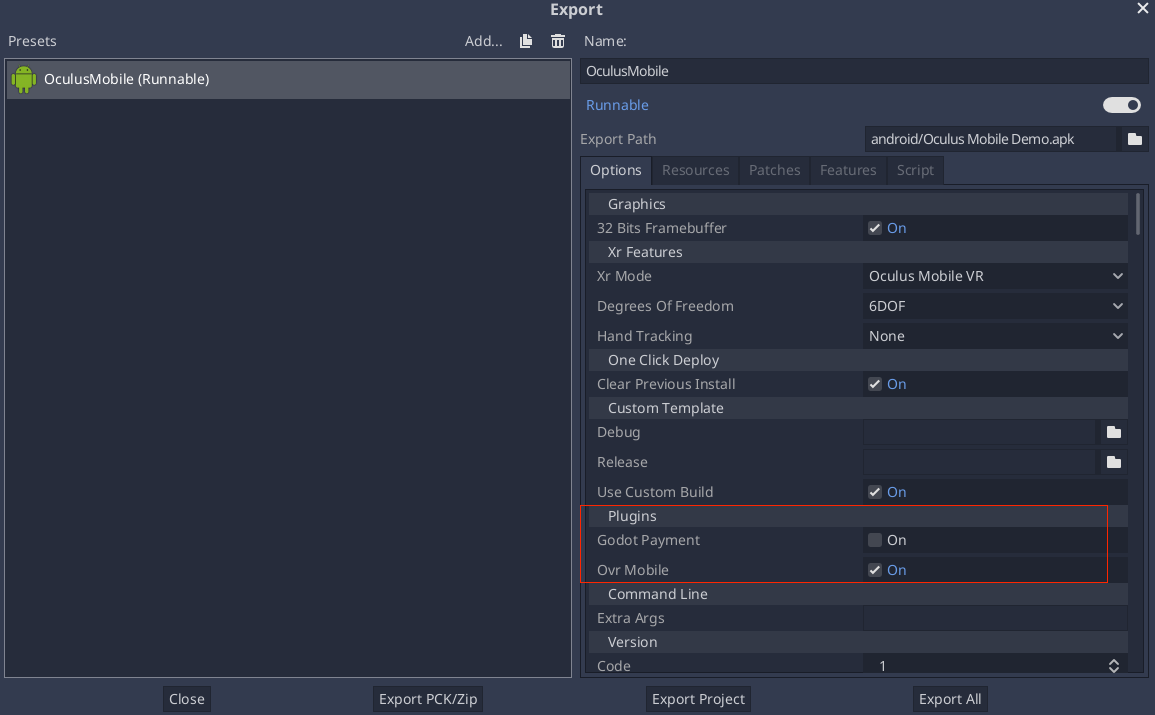
From your script:
if Engine.has_singleton("MyPlugin"):
var singleton = Engine.get_singleton("MyPlugin")
print(singleton.myPluginFunction("World"))
Bundling GDExtension resources¶
An Android plugin can define and provide C/C++ GDExtension resources, either to provide and/or access functionality from the game logic.
The GDExtension resources can be bundled within the plugin aar
file which simplifies the distribution and deployment process:
The shared libraries (
.so
) for the defined GDExtension libraries will be automatically bundled by theaar
build system.Godot
*.gdnlib
和*.gdns
资源文件必须在插件assets
目录下手动定义. 这些资源相对于assets
目录的推荐路径应该为godot/plugin/v1/[PluginName]/
.
For GDExtension libraries, the plugin singleton object must override the org.godotengine.godot.plugin.GodotPlugin::getPluginGDNativeLibrariesPaths()
method,
and return the paths to the bundled GDExtension libraries config files (*.gdextension
). The paths must be relative to the assets
directory.
At runtime, the plugin will provide these paths to Godot core which will use them to load and initialize the bundled GDExtension libraries.
参考实现¶
故障排除¶
Godot 在加载时崩溃¶
检查 adb logcat
是否存在可能的问题,然后:
检查插件暴露的方法是否使用了下列 Java 类型:
void
、boolean
、int
、float
、java.lang.String
、org.godotengine.godot.Dictionary
、int[]
、byte[]
、float[]
、java.lang.String[]
。暂时不支持更复杂的数据类型.